Getting Started with Safaricom Daraja API: A Guide for Developers

Safaricom’s Daraja API enables developers to integrate M-Pesa, the leading mobile money platform in Kenya, into their applications. Whether you’re building an e-commerce website, a mobile app, or a custom payment system, the Daraja API offers a flexible and reliable way to handle payments. This guide walks you through the basics of setting up and using the Daraja API.
Key Features of the Safaricom Daraja API
- C2B (Customer to Business): Allows customers to make payments to your business via M-Pesa.
- B2C (Business to Customer): Enables businesses to disburse payments to customers.
- B2B (Business to Business): Facilitates payments between businesses.
- Lipa na M-Pesa Online: Ideal for e-commerce, allowing real-time payments.
- Balance Inquiry and Transaction Status: Check account balances and track payments.
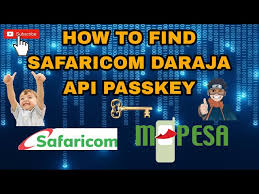
Step-by-Step Integration Guide
1. Register for a Safaricom Developer Account
- Visit the Safaricom Developer Portal and create an account.
- Log in to your account and access the Daraja API dashboard.
2. Create an Application
- Click on “My Apps” and select “Create a New App.”
- Provide a name for your application and select the APIs you want to use (e.g., M-Pesa Sandbox).
- Save your application to generate the Consumer Key and Consumer Secret for API authentication.
3. Set Up Your Development Environment
To interact with the Daraja API, you’ll need:
- API Keys: Obtain your Consumer Key and Consumer Secret from the app you created.
- Environment: Use the Sandbox environment for testing and the Production environment for live transactions.
- HTTP Client: Tools like Postman or programming libraries (e.g., Python requests, PHP cURL) for sending API requests.
4. Obtain an Access Token
Use the Consumer Key and Consumer Secret to get an access token. Here’s an example in PHP:
function getAccessToken() {
$consumerKey = 'your_consumer_key';
$consumerSecret = 'your_consumer_secret';
$credentials = base64_encode($consumerKey . ':' . $consumerSecret);
$ch = curl_init('https://sandbox.safaricom.co.ke/oauth/v1/generate?grant_type=client_credentials');
curl_setopt($ch, CURLOPT_HTTPHEADER, ['Authorization: Basic ' . $credentials]);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = json_decode(curl_exec($ch));
curl_close($ch);
return $response->access_token;
}
5. Implement M-Pesa API Endpoints
Below are common use cases:
a. C2B (Customer to Business) Payments
Use this API to process payments from customers to your business:
- Register your callback URLs using the
RegisterURL
API. - Use the
Simulate
API for testing payment requests in the sandbox.
b. Lipa na M-Pesa Online
Ideal for real-time payments on websites or apps:
- Make a request to the
STKPush
API to initiate a payment. - Monitor the callback URL for the payment response.
Sample STK Push request:
function lipaNaMpesa($accessToken) {
$url = 'https://sandbox.safaricom.co.ke/mpesa/stkpush/v1/processrequest';
$data = [
'BusinessShortCode' => '174379',
'Password' => base64_encode('BusinessShortCode' . 'PassKey' . 'Timestamp'),
'Timestamp' => 'YYYYMMDDHHMMSS',
'TransactionType' => 'CustomerPayBillOnline',
'Amount' => '10',
'PartyA' => '2547XXXXXXXX',
'PartyB' => '174379',
'PhoneNumber' => '2547XXXXXXXX',
'CallBackURL' => 'https://yourdomain.com/callback',
'AccountReference' => 'Invoice123',
'TransactionDesc' => 'Payment for goods',
];
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_HTTPHEADER, ['Authorization: Bearer ' . $accessToken]);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
return $response;
}
Best Practices
- Secure Your Credentials: Never expose your Consumer Key, Consumer Secret, or access tokens publicly.
- Use HTTPS: Ensure your endpoints and callback URLs are secured with SSL.
- Monitor API Limits: Be mindful of API request limits to avoid throttling.
- Test Thoroughly: Use the sandbox environment to simulate transactions before going live.
- Handle Errors Gracefully: Implement robust error-handling mechanisms for API failures.
Conclusion
The Safaricom Daraja API opens up numerous possibilities for businesses to integrate seamless payment solutions into their platforms. By following this guide, you can confidently set up the API and start processing transactions with M-Pesa.
Have questions or need help? Drop a comment below, and let’s get you started with M-Pesa integration!
And if you need assistance in integrating M-pesa to your website contact me through my site, Almalick Kweyu